1. Introduction
What is the Importance of List in Java?
In Java programming, “List” is a data structure that appears very frequently. Especially in situations where you want to manage multiple values together, it is more flexible and easier to use than arrays, making it highly valued in many practical scenarios.
“List” is a core interface in the Java Collections Framework and provides a mechanism to handle various situations through different implementation classes such as ArrayList and LinkedList. The ability to perform operations like adding, deleting, searching, and updating data intuitively is one of the reasons why List is favored.
Purpose and Target Audience of This Article
This article will systematically explain “Java List” from basics to advanced topics in an easy-to-understand way for beginners. The main target audience is as follows:
- Those who are just starting to learn Java and are unsure about how to use List
- Those who want to clearly understand the difference between Array and List
- Those who are struggling with choosing between ArrayList and LinkedList
- Those who want to review the basics before using List in practice
By the time you finish reading this article, our goal is for you to gain a solid understanding of the basic concepts, implementation methods, and specific operations of List in Java, enabling you to code with confidence.
From the next chapter, we will start by explaining the basic part, “What is List?”, step by step.
2. What is List?
Overview and Characteristics of List
“List” in Java is a collection interface that holds elements in an ordered sequence. Its biggest features are that the order of element addition is preserved and that individual elements can be accessed using an index (starting from 0).
List is provided as part of the Collections Framework and has the following features:
- Allows duplicate elements
- Can get, update, and delete elements by specifying an index
- Can dynamically increase or decrease the number of elements (unlike arrays, it is not fixed-size)
This allows for flexible data manipulation and is very frequently used in practical work.
Difference from Array
In Java, arrays (like int[]
or String[]
) also exist as a means to hold multiple values, but there are several differences from List.
Comparison Item | Array | List |
---|---|---|
Changing number of elements | Not possible (fixed-size) | Possible (can increase/decrease dynamically) |
Provided functionality | Minimal operations (indexed access, length retrieval) | Rich methods (add, remove, contains, etc.) |
Type | Can handle primitive types | Object types only (wrapper classes required) |
Type safety | Arrays checked at compile time | Can strictly specify type with Generics |
As such, List is a more flexible and feature-rich collection, making it more practical than arrays in many situations.
List Interface and its Implementation Classes
When using List in Java, you generally declare a variable using the List
interface and create an instance with a specific class (implementation class). Representative implementation classes are as follows:
- ArrayList
Similar structure to an array, allowing fast access. Strong for searching data and random access. - LinkedList
Implemented with a doubly linked list structure. Fast for insertion and deletion, suitable for lists where operations are frequent. - Vector
Similar to ArrayList but slightly heavier as it is thread-safe. Not used much nowadays.
Generally, ArrayList is the most commonly used unless there is a special reason. It’s good to choose the appropriate one based on the performance comparison described later, depending on the use case.
3. Basic Usage of List
This section explains the basic operations when using List in Java step by step. Here, we will primarily use ArrayList as an example to introduce the representative operations of List.
Declaration and Initialization of List
First, let’s look at the basic declaration and initialization of a List using ArrayList
.
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> fruits = new ArrayList<>();
}
}
It is common practice to declare a variable with the List
interface and instantiate it with ArrayList
. Generics are used to specify the type to be stored (here, String
).
Adding Elements (add)
To add elements to a List, use the add()
method.
fruits.add("apple");
fruits.add("banana");
fruits.add("orange");
This adds three elements to the List in sequence. List preserves the order of addition.
Getting Elements (get)
To get an element at a specified index, use get(int index)
.
System.out.println(fruits.get(0)); // "apple" will be displayed
Note that indices start from 0.
Updating Elements (set)
To update an element at a certain position, use set(int index, E element)
.
fruits.set(1, "grape"); // The second element "banana" is replaced with "grape"
Removing Elements (remove)
You can also remove elements by a specific index or the element itself.
fruits.remove(0); // Removes the first element
fruits.remove("orange"); // Removes "orange" (only the first match)
Getting List Size (size)
The current number of elements can be obtained with the size()
method.
System.out.println(fruits.size()); // Returns 2, etc.
Checking for Element Existence (contains)
To check if a specific element is included in the List, use contains()
.
if (fruits.contains("grape")) {
System.out.println("grape is present");
}
Summary: List of Frequently Used Basic Operations
Operation | Method Example | Description |
---|---|---|
Addition | add("element") | Adds to the end |
Retrieval | get(index) | References an element |
Update | set(index, new element) | Changes the element at the specified position |
Removal | remove(index/element) | Removes the specified element |
Get Size | size() | Gets the number of elements |
Check Existence | contains("element") | Checks if a specific element exists |
4. List Operation Examples
In this chapter, we will introduce practical operation examples using Java’s List. There are many situations where you want to process elements in a list sequentially, and here we will cover representative methods using for loop, enhanced for loop, and Stream API.
Iteration using a for loop
The most basic method is to retrieve elements using an index within a for
loop.
List<String> fruits = new ArrayList<>();
fruits.add("apple");
fruits.add("banana");
fruits.add("orange");
for (int i = 0; i < fruits.size(); i++) {
System.out.println(fruits.get(i));
}
This method allows for fine-grained control using the index. For example, it is effective when you want to process only elements at even indices.
Iteration using an enhanced for loop (for-each)
If you want to process all elements sequentially without worrying about the index, the enhanced for loop is convenient.
for (String fruit : fruits) {
System.out.println(fruit);
}
The syntax is simple and easy to read, making it one of the most commonly used methods. This is sufficient for simple processing.
Iteration using Lambda Expressions and Stream API
Since Java 8, you can also use the syntax with Stream API and lambda expressions.
fruits.stream().forEach(fruit -> System.out.println(fruit));
The strength of this notation is that multiple processes can be chained together. For example, you can easily filter and then print elements based on specific criteria.
fruits.stream()
.filter(fruit -> fruit.contains("a"))
.forEach(System.out::println);
In this example, it prints only fruits that contain “a”. This is especially recommended for those who want to get used to functional style coding.
Choosing the Right Method
Method | Advantages | Suitable Situations |
---|---|---|
Regular for loop | Allows index control | Processing that requires element numbers |
Enhanced for loop | Simple and easy to read syntax | Simple iteration processing |
Stream API | Strong for conditional and chained processing | When combining filtering, mapping, and reduction |
5. Differences and Usage of ArrayList and LinkedList
The representative classes that implement Java’s List interface are ArrayList and LinkedList. Both can be used as List in the same way, but they have differences in internal structure and performance characteristics, so it is important to use them appropriately in the right situations.
Characteristics and Suitable Use Cases of ArrayList
ArrayList
internally uses a dynamic array (resizable array).
Main Characteristics:
- Very fast for random access (index-based)
- Adding elements at the end of the list is fast (average O(1))
- Insertion and deletion in the middle are slower (O(n))
Suitable Situations:
- Situations where searching (
get()
) is frequent - Situations where the number of elements can be predicted to some extent beforehand
- Processing where element addition/deletion is minimal, focusing on reading
List<String> list = new ArrayList<>();
Characteristics and Suitable Use Cases of LinkedList
LinkedList
is implemented with a doubly linked list structure.
Main Characteristics:
- Fast for adding and deleting elements (especially at the beginning or end)
- Random access (
get(index)
) is slow (O(n)) - Memory consumption is slightly higher than ArrayList
Suitable Situations:
- Situations where elements are frequently inserted or deleted (especially at the beginning or in the middle)
- When you want to use it like a Queue or Stack
- When focusing on iteration and index access is not needed
List<String> list = new LinkedList<>();
Performance Comparison
The following table shows the theoretical time complexity (Big O notation) for commonly used operations.
Operation | ArrayList | LinkedList |
---|---|---|
get(int index) | O(1) | O(n) |
add(E e) (at the end) | O(1) | O(1) |
add(int index, E e) | O(n) | O(n) |
remove(int index) | O(n) | O(n) |
Iteration | O(n) | O(n) |
* Actual processing time can also be affected by data size, JVM optimization, etc.
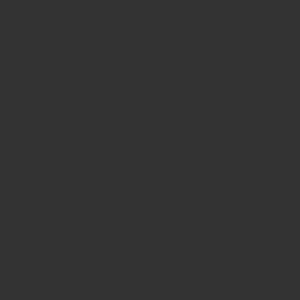
Points for Practical Usage Differentiation
- If you are treating data as a list and accessing by index, use ArrayList
- If insertion/deletion at the beginning or in the middle is frequent, use LinkedList
- For performance-sensitive processing, always benchmark and verify
6. Advanced Usage of List
Here, we will introduce advanced techniques to use Java’s List even more conveniently. List can perform various operations not only as a simple collection of data but also through sorting, shuffling, filtering, transformation, etc.
Sorting a List (Collections.sort)
Using Collections.sort()
, you can sort the elements in a List in ascending order. Elements must implement the Comparable
interface.
import java.util.*;
List<String> fruits = new ArrayList<>();
fruits.add("banana");
fruits.add("apple");
fruits.add("orange");
Collections.sort(fruits);
System.out.println(fruits); // [apple, banana, orange]
Sorting in a custom order (using Comparator)
fruits.sort(Comparator.reverseOrder()); // Sorts in descending order
Shuffling a List (Collections.shuffle)
To randomly reorder the elements, you can use Collections.shuffle()
.
Collections.shuffle(fruits);
System.out.println(fruits); // [banana, orange, apple] (example)
This is useful when you want a deck of cards for a game or a random display order.
Filtering using Stream API (filter)
Using Stream
from Java 8 onwards, you can concisely write code to extract only elements that match a condition.
List<String> filtered = fruits.stream()
.filter(fruit -> fruit.contains("a"))
.collect(Collectors.toList());
System.out.println(filtered); // [apple, banana, orange] (depending on original content and filter)
Transformation using Stream API (map)
To transform elements into a different format, use map()
.
List<Integer> lengths = fruits.stream()
.map(String::length)
.collect(Collectors.toList());
System.out.println(lengths); // Lengths of each fruit name [5, 6, 6] etc.
map()
is a powerful tool for data format conversion and preprocessing.
Advanced Operations Summary
Operation | Usage Example | Main Use Cases |
---|---|---|
Sort | Collections.sort(list) | Sort in ascending order |
Shuffle | Collections.shuffle(list) | Randomize the order of elements |
Filter | stream().filter(...).collect() | Extract only elements that match a condition |
Transform | stream().map(...).collect() | Transform the type or value of elements |
7. Common Errors and Their Solutions
When working with Lists in Java, one of the things beginners often stumble upon is “exceptions (errors)”. Here, we will specifically explain representative errors that occur frequently, their causes, and how to solve them.
IndexOutOfBoundsException
Cause:
Occurs when trying to access a non-existent index.
List<String> list = new ArrayList<>();
list.add("apple");
System.out.println(list.get(1)); // Error: Index 1 out of bounds
Solution:
Check the size before accessing or control access with conditional branching to ensure the index is valid.
if (list.size() > 1) {
System.out.println(list.get(1));
}
NullPointerException
Cause:
Occurs when calling a method on a List or a List element that is null
.
List<String> list = null;
list.add("apple"); // NullPointerException occurs
Solution:
Check beforehand that the variable is not null, or utilize Optional, etc.
if (list != null) {
list.add("apple");
}
Also, be careful about forgetting to initialize:
List<String> list = new ArrayList<>(); // Correct initialization
ConcurrentModificationException
Cause:
Occurs when the List is directly modified while iterating through it using a for-each
loop or Iterator
.
for (String fruit : list) {
if (fruit.equals("banana")) {
list.remove(fruit); // ConcurrentModificationException
}
}
Solution:
Use an Iterator
to safely remove elements, or use methods like removeIf()
.
Iterator<String> it = list.iterator();
while (it.hasNext()) {
if (it.next().equals("banana")) {
it.remove(); // Safe removal
}
}
Or, more concisely from Java 8 onwards:
list.removeIf(fruit -> fruit.equals("banana"));
Other Points to Note
- Checking that the List is not null
- It is very common to declare a variable but not use it. Initialization is essential.
- Understanding that indices start from 0
- Beginners often mistakenly think “the first element is index 1”.
Error Countermeasures Summary
Error Name | Primary Cause | Example Solutions |
---|---|---|
IndexOutOfBoundsException | Accessing a non-existent index | Check length with size() |
NullPointerException | List or element is null | Don’t forget initialization, perform null checks |
ConcurrentModificationException | Directly modifying the List during iteration | Operate with Iterator or utilize removeIf() |
8. Conclusion
Reviewing the Basics of Java List
In this article, we have explained the basics to advanced aspects of List in Java step by step. List is particularly frequently used among Java collections and is an important tool for flexibly handling data.
First, after understanding what List is, we learned the following points:
- List is an ordered collection that allows duplicates and supports index operations
- There are representative implementation classes such as ArrayList and LinkedList, each with different characteristics and use cases
- Mastering basic operations (add, get, update, remove, search) allows for flexible data manipulation
- Iterative processing suitable for the situation, such as for loops, enhanced for loops, and Stream API
- Supports advanced operations such as sorting, filtering, and transformation
- Understanding common errors, their causes, and solutions helps prevent issues
Differentiating between ArrayList and LinkedList Usage
Choosing which List implementation to use is important and should be based on the content of the processing and the amount of data. The following criteria can serve as a guide:
- ArrayList: Frequent random access, primarily reading
- LinkedList: Frequent insertion/deletion, order of access is important
Towards Future Learning
List is just the “entry point” to Java’s collections. To handle more advanced data structures and utilities, it is recommended to deepen your understanding of the following classes and features:
- Set and Map: Managing unique elements, key-value pair structure
- Collections utility class: Sorting, finding min/max, etc.
- Utilizing Stream API: Introducing functional programming
- Understanding Generics: Type-safe collection operations
Mastering the basics of List will make your overall Java programming significantly easier to manage.
Frequently Asked Questions (FAQ)
We have compiled points that beginners often have questions about regarding Java’s List. We have selected content that is frequently encountered in practice.
Q1. What is the difference between Java’s List and Array?
A. An array has a fixed number of elements and its size must be determined at declaration. On the other hand, a List has a variable size, allowing for flexible addition and deletion of elements. Furthermore, List comes with many convenient methods (add
, remove
, contains
, etc.) and is superior in terms of readability and maintainability.
Q2. Which should I use, ArrayList or LinkedList?
A. ArrayList
is suitable mainly when there is frequent random access (retrieval by index). LinkedList
is suitable when element insertion and deletion occur frequently. When in doubt, starting with ArrayList
is generally recommended.
Q3. Can I store primitive types (like int or double) in a List?
A. Not directly. Since Java’s List handles only object types, for primitive types like int
, you need to use their corresponding wrapper classes (Integer
, Double
, etc.).
List<Integer> numbers = new ArrayList<>();
numbers.add(10); // Auto-boxed and stored as Integer type
Q4. How can I sort the elements in a List?
A. You can sort in ascending order using Collections.sort(list)
. Also, if you want to sort in a custom order, you can specify a Comparator
for flexible ordering.
Q5. What should I do if I want to manage elements without duplicates?
A. List is a collection that allows duplicates. If you want to avoid duplicates, consider using a Set
(e.g., HashSet
). However, note that the order is not guaranteed. If you want to remove duplicates while keeping it a List, the following Stream processing is also possible:
List<String> distinctList = list.stream()
.distinct()
.collect(Collectors.toList());
Q6. What do I do when I want to clear all elements from a List?
A. You can remove all elements from a List using the clear()
method.
list.clear();
Q7. What are the most frequently used operations in List?
A. The most frequently used operations in practice are add
(addition), get
(retrieval), remove
(deletion), and size
(getting size). Mastering these will cover most basic processing.